GestureModel Interface Reference
GestureModel is an interface for classes that attempt to serve as a model structure for gestures. More...

Public Member Functions | |
boolean | addAccel (int inum, Accel3D acc) |
Adds an additional Accel3D to the end of a gesture instance. | |
void | addInstance (Vector< Accel3D > instance) |
Adds an entire gesture instance to the gesture set. | |
void | clearStats () |
Clears all saved statistics associated with this GestureModel. | |
void | correct () |
Signifies a correct recognition event to the GestureModel. | |
double | getAverageRecognitionProbability () |
Returns the average certainty currently associated with this GestureModel. | |
double | getDefaultProbability () |
Returns a value that represents the default probability associated with this GestureModel. | |
BufferedImage | getIcon () |
Returns the icon of this gesture as a BufferedImage. | |
String | getName () |
Returns the name associated with this GestureModel. | |
int | getNumCorrect () |
Accessor for the number correct statistic. | |
int | getNumIncorrect () |
Accessor for the number incorrect statistic. | |
int | getNumNotRecognized () |
Accessor for the number not recognized statistic. | |
double | getProbability (Vector< Accel3D > instance) |
Computes and returns the probability with which the given gesture instance matches with this GestureModel. | |
int | getType () |
Accessor for the GestureModel type index. | |
Vector< Vector< Accel3D > > | getTrainingSet () |
Accessor for the training set of gesture instances associated with this GestureModel. | |
void | incorrect () |
Signifies an incorrect recognition event to the GestureModel. | |
void | matchedWithProbability (double prob) |
Signifies a recognition event that occurred with a given probability. | |
void | notRecognized () |
Signifies an unrecognized recognition event to the GestureModel. | |
boolean | removeAccel (int inum, int anum) |
Removes an acceleration value from the gesture set. | |
boolean | removeInstance (int inum) |
Removes a gesture instance from the gesture set. | |
void | setIcon (byte[] imageBuffer) throws IOException |
Associates a byte array image with the GestureModel. | |
void | setName (String n) |
Associates a representative name with this GestureModel. | |
boolean | train () |
Trains the GestureModel, constructing everything necessary for recognition to take place. | |
Static Public Attributes | |
static final int | GESTUREHMM = 0 |
This is the GestureModel type index for GestureHMM models. | |
static final int | NUM_TYPES_SUPPORTED = 1 |
This is the number of GestureModel implementations currently supported by GestureModel. |
Detailed Description
GestureModel is an interface for classes that attempt to serve as a model structure for gestures.
"Gestures" are understood in terms of acceleration data. In particular, GestureModel is based on a 3D-acceleration based approach to reading gestures. GestureModel's must be able to train with data, recognize against gesture instances, as well as record statistics relevant to measuring effectiveness of training/recognition.
Member Function Documentation
boolean addAccel | ( | int | inum, | |
Accel3D | acc | |||
) |
Adds an additional Accel3D to the end of a gesture instance.
- Parameters:
-
inum The index of the gesture instance to modify acc The Accel3D to insert
- Returns:
- a boolean signifying success or failure of the insertion
Implemented in GestureHMM.
void addInstance | ( | Vector< Accel3D > | instance | ) |
Adds an entire gesture instance to the gesture set.
- Parameters:
-
instance The instance to insert
Implemented in GestureHMM.

void clearStats | ( | ) |
Clears all saved statistics associated with this GestureModel.
Implemented in GestureHMM.
void correct | ( | ) |
Signifies a correct recognition event to the GestureModel.
Statistics for the model are updated accordingly.
Implemented in GestureHMM.

double getAverageRecognitionProbability | ( | ) |
Returns the average certainty currently associated with this GestureModel.
The average certainty is calculated based on all calls made to frog.GestureModel#matchedWithProbability(double).
- Returns:
- the average recognition probability (certainty)
Implemented in GestureHMM.
double getDefaultProbability | ( | ) |
Returns a value that represents the default probability associated with this GestureModel.
This is useful, for example, in Bayesian classification.
- Returns:
- the default probability of this model.
Implemented in GestureHMM.
BufferedImage getIcon | ( | ) |
Returns the icon of this gesture as a BufferedImage.
- Returns:
- the icon of this gesture; or
null
if the gesture has no icon.
Implemented in GestureHMM.

String getName | ( | ) |
Returns the name associated with this GestureModel.
(e.g. a model for a circle might appropriately be named "Circle")
- Returns:
- the name of the GestureModel.
Implemented in GestureHMM.
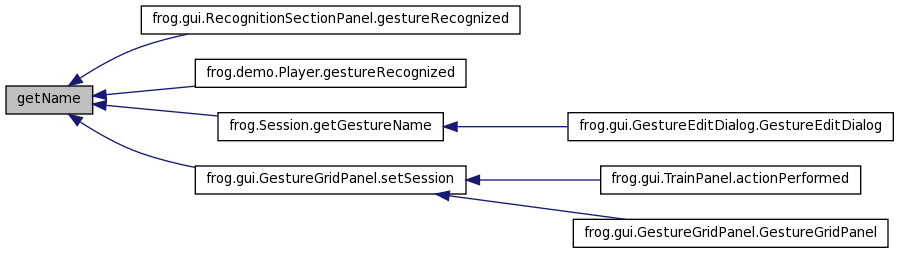
int getNumCorrect | ( | ) |
Accessor for the number correct statistic.
This value is entirely dependent on calls to frog.GestureModel#correct()
- Returns:
- the saved number of correct recognition events.
Implemented in GestureHMM.

int getNumIncorrect | ( | ) |
Accessor for the number incorrect statistic.
This value is entirely dependent on calls to frog.GestureModel#incorrect()
- Returns:
- the saved number of incorrect recognition events.
Implemented in GestureHMM.

int getNumNotRecognized | ( | ) |
Accessor for the number not recognized statistic.
This value is entirely dependent on calls to frog.GestureModel#notRecognized()
- Returns:
- the saved number of unrecognized recognition events.
Implemented in GestureHMM.

double getProbability | ( | Vector< Accel3D > | instance | ) |
Computes and returns the probability with which the given gesture instance matches with this GestureModel.
- Parameters:
-
instance the series of acceleration vectors representing a single gesture instance
- Returns:
- the probability that the instance matches this model
Implemented in GestureHMM.
Vector<Vector<Accel3D> > getTrainingSet | ( | ) |
Accessor for the training set of gesture instances associated with this GestureModel.
- Returns:
- the model's gesture set
Implemented in GestureHMM.

int getType | ( | ) |
Accessor for the GestureModel type index.
Should return the value as specified in the GestureModel interface.
- Returns:
- the GestureModel type index.
Implemented in GestureHMM.
void incorrect | ( | ) |
Signifies an incorrect recognition event to the GestureModel.
Statistics for the model are updated accordingly.
Implemented in GestureHMM.

void matchedWithProbability | ( | double | prob | ) |
Signifies a recognition event that occurred with a given probability.
This updates the average recognition probability of the GestureModel, as accessed by frog.GestureModel#getAverageRecognitionProbability()
- Parameters:
-
prob the probability (0-1) with which the match occurred
Implemented in GestureHMM.

void notRecognized | ( | ) |
Signifies an unrecognized recognition event to the GestureModel.
Statistics for the model are updated accordingly.
Implemented in GestureHMM.

boolean removeAccel | ( | int | inum, | |
int | anum | |||
) |
Removes an acceleration value from the gesture set.
- Parameters:
-
inum the index of the instance to access anum the index of the acceleration to remove
- Returns:
- a boolean signifying success or failure of the deletion
Implemented in GestureHMM.

boolean removeInstance | ( | int | inum | ) |
Removes a gesture instance from the gesture set.
- Parameters:
-
inum the index of the instance to remove
- Returns:
- a boolean signifying success or failure of the deletion
Implemented in GestureHMM.

void setIcon | ( | byte[] | imageBuffer | ) | throws IOException |
Associates a byte array image with the GestureModel.
- Parameters:
-
imageBuffer the byte array image
- Exceptions:
-
IOException thrown if image reading fails
Implemented in GestureHMM.

void setName | ( | String | n | ) |
Associates a representative name with this GestureModel.
- Parameters:
-
n the name to associate
Implemented in GestureHMM.
boolean train | ( | ) |
Trains the GestureModel, constructing everything necessary for recognition to take place.
Implemented in GestureHMM.
Member Data Documentation
final int GESTUREHMM = 0 [static] |
This is the GestureModel type index for GestureHMM models.
final int NUM_TYPES_SUPPORTED = 1 [static] |
This is the number of GestureModel implementations currently supported by GestureModel.
The documentation for this interface was generated from the following file:
- /Users/dev/Documents/SVN brazos.cs.tcu.edu/trunk/FROG/src/frog/GestureModel.java