DemoController Class Reference
The DemoController manages all Sprites and sound in the game. More...

Public Member Functions | |
DemoController (DemoGame game) | |
Creates a new DemoController for the instance of DemoGame. | |
final void | draw (Graphics g) |
Calls the draw method on all sprites after painting the background. | |
void | killUFO (int type, int player) |
Creates a new KillEvent for a given player on a given UFO. | |
final void | update () |
Calls the update method of each Sprite which should in turn move one step across the screen. | |
Static Public Attributes | |
static final int | CIRCLE = 0 |
Shape recognized in this game. | |
static final int | SQUARE = 1 |
static final int | TRIANGLE = 2 |
static final int | X = 3 |
static final int | Z = 4 |
Protected Member Functions | |
void | notifyAbduction (CowSprite cow) |
Allows the controller to put the abducted cow to a special queue. | |
void | notifyDeadUFO () |
A UFO has finished exploding and needs to be removed from the ufoDeath queue. | |
void | notifyRelease (CowSprite cow) |
Notifies the controller of the release of a cow from the hold of a UFO. | |
void | removeCow (CowSprite cow) |
Completely removes the given CowSprite from the game. | |
void | removeUFO (UFOSprite ufo) |
Removes a UFO from the game and decrements the total UFO count by 1. | |
Package Attributes | |
boolean | enableSound = true |
Determines if sound should be enabled or not. |
Detailed Description
The DemoController manages all Sprites and sound in the game.
After the initial DemoGame window has been set up, it uses DemoController to run the game. After DemoController has been created, DemoGame's only job will be to ensure that the requested FPS is maintained and that special keyboard events are handled (such as mute).
Constructor & Destructor Documentation
DemoController | ( | DemoGame | game | ) |
Creates a new DemoController for the instance of DemoGame.
- Parameters:
-
game the reference to the DemoGame that this controller is a part of
Member Function Documentation
final void draw | ( | Graphics | g | ) |
Calls the draw method on all sprites after painting the background.
- Parameters:
-
g the Graphics context to draw to.
void killUFO | ( | int | type, | |
int | player | |||
) |
Creates a new KillEvent for a given player on a given UFO.
A KillEvent will be executed on the next update and if a matching UFO is found to the type given, it will be deleted and points awarded to that player.
- Parameters:
-
type the type of UFO to destroy. Could be CIRCLE, TRIANGLE, etc. player the Player to give the kill points to.
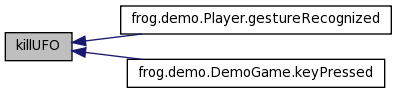
void notifyAbduction | ( | CowSprite | cow | ) | [protected] |
Allows the controller to put the abducted cow to a special queue.
A cow on that queue will not be handed out as a target to the other UFOs. All of that cow's abductors will be given new cows to chase after.
- Parameters:
-
cow the CowSprite that needs to be modified.


void notifyDeadUFO | ( | ) | [protected] |
A UFO has finished exploding and needs to be removed from the ufoDeath queue.

void notifyRelease | ( | CowSprite | cow | ) | [protected] |
Notifies the controller of the release of a cow from the hold of a UFO.
A released cow will be moved back to the available list of cows and all UFOs are assigned new targets.
- Parameters:
-
cow the CowSprite that was released.

void removeCow | ( | CowSprite | cow | ) | [protected] |
void removeUFO | ( | UFOSprite | ufo | ) | [protected] |
Removes a UFO from the game and decrements the total UFO count by 1.
- Parameters:
-
ufo the UFOSprite to destroy.

final void update | ( | ) |
Calls the update method of each Sprite which should in turn move one step across the screen.
Every update checks for game over condition and occasionally makes the cows "moo".


Member Data Documentation
final int CIRCLE = 0 [static] |
Shape recognized in this game.
boolean enableSound = true [package] |
Determines if sound should be enabled or not.
final int SQUARE = 1 [static] |
final int TRIANGLE = 2 [static] |
final int X = 3 [static] |
final int Z = 4 [static] |
The documentation for this class was generated from the following file:
- /Users/dev/Documents/SVN brazos.cs.tcu.edu/trunk/FROG/src/frog/demo/DemoController.java